Building a Charity Search App in C# Using the OrgHunter Charity API
Learn how to use the OrgHunter Charity API to build a C# app that searches for charities by name, city, or state, with easy setup and integration steps.
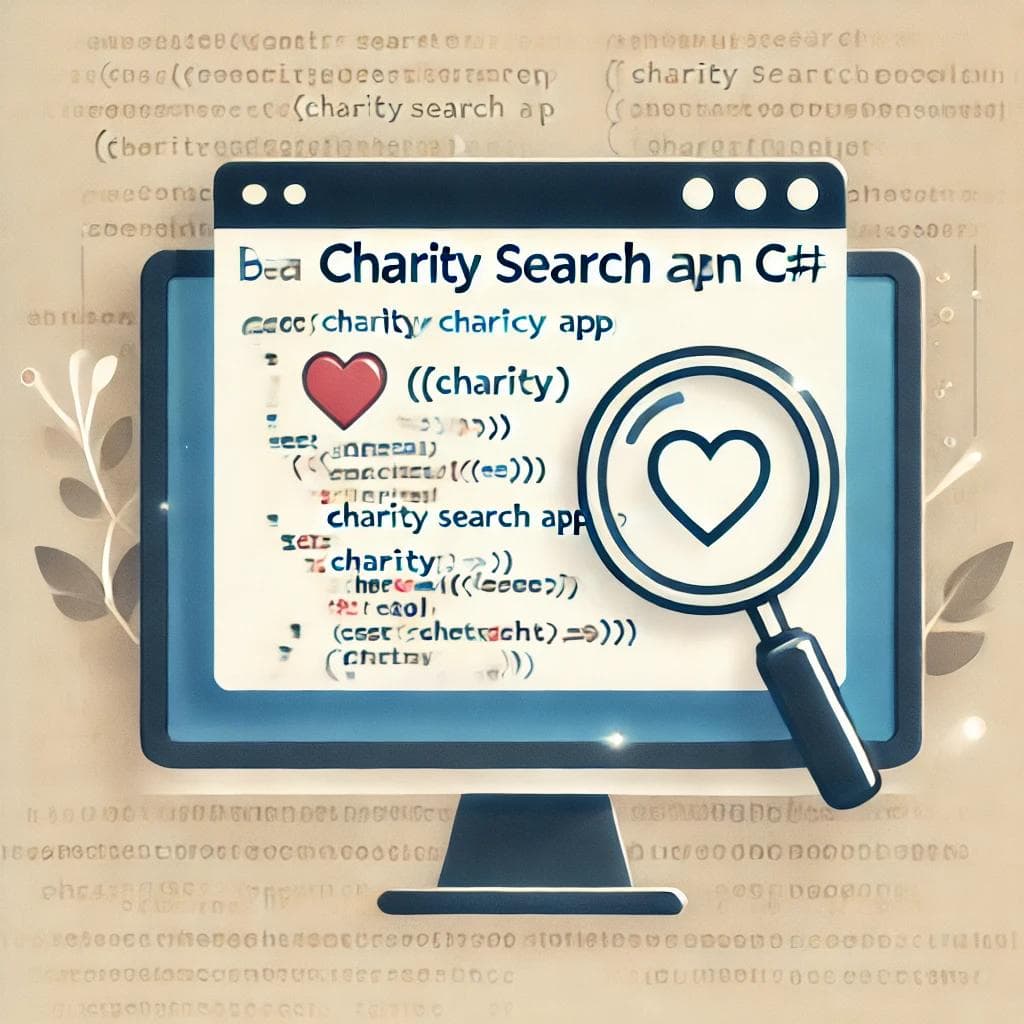
C# Charity API Tutorial: Find Nonprofits with OrgHunter (with Code Examples)
Want to empower your users to find and support their favorite charities? Need to access reliable charity data for your application? A charity API can help! In this tutorial, we'll show you how to easily use the OrgHunter Charity API to find and access information on millions of US-based nonprofits. We'll walk you through a C# code example so you can see how simple it is to integrate charity data into your projects.
Why Use a Charity API?
Integrating a charity API offers several benefits for developers:
- Real-time data: Access up-to-date information on charities, including their legal name, EIN, address, and mission.
- Simplified search: Easily search for charities by name, location, cause area, or EIN.
- Streamlined giving: Enable users to donate to charities directly within your application.
- Enhanced user experience: Provide users with valuable information and tools to support the causes they care about.
Introducing OrgHunter's Charity API
OrgHunter's Charity API is a powerful tool that provides comprehensive data on millions of US-based charities. With OrgHunter, you can:
- Verify charity status: Ensure that the organizations in your database are legitimate and eligible to receive donations.
- Access detailed information: Retrieve comprehensive profiles of charities, including their mission, programs, and financials.
- Simplify grantmaking: Streamline the process of awarding and tracking grants.
Getting Started
Before we dive into the code, you'll need an API key. To get started, sign up for a free account on the OrgHunter website. The OrgHunter Charity API Documentation [invalid URL removed] page provides detailed API documentation and code examples.
C# Code Example: Building a Charity Search Application
Let's create a simple C# console application that searches for charities using the OrgHunter API. Here's the code:
Code snippet
using System;
using System.Net.Http;
using System.Threading.Tasks;
namespace CharitySearchApp
{
class Program
{
private static async Task SearchCharities(string searchTerm)
{
// Replace "yourKey" with your actual OrgHunter API key
string apiKey = "yourKey";
string url = $"http://data.orghunter.com/v1/charitysearch?user_key={apiKey}&searchTerm={searchTerm}";
using (HttpClient client = new HttpClient())
{
HttpResponseMessage response = await client.PostAsync(url, null);
if (response.IsSuccessStatusCode)
{
string responseBody = await response.Content.ReadAsStringAsync();
// Process the JSON response here. You can use a library like Newtonsoft.Json
// to deserialize the response and access specific data fields.
Console.WriteLine(responseBody);
}
else
{
Console.WriteLine($"Error: {response.StatusCode}");
}
}
}
static async Task Main(string[] args)
{
await SearchCharities("humane society");
}
}
}
Code Explanation:
SearchCharities
Function: This function sends a POST request to the OrgHunter Charity API using the provided search term.- It constructs the API URL using your API key and the search term.
- It uses
HttpClient
to send the request and retrieve the response. - It checks if the request was successful (
IsSuccessStatusCode
) and then reads the response body.
Main
function: This function calls theSearchCharities
function with the search term "humane society."
Handling the API Response:
The API response will be in JSON format. You can use a library like Newtonsoft.Json to deserialize the JSON and access the data you need. For example, to get the name of the first charity in the results:
Code snippet
// ... (previous code) ...
string responseBody = await response.Content.ReadAsStringAsync(); // Deserialize the JSON response dynamic jsonResponse = JsonConvert.DeserializeObject(responseBody);
// Access the name of the first charity
string charityName = jsonResponse.data.results[0].charityName;
Console.WriteLine($"Charity Name: {charityName}");
// ... (rest of the code) ...
Use Cases and Benefits
Here are a few examples of how you can use the OrgHunter Charity API in your applications:
- Charity search tool: This tool allows users to search for charities by name, location, or cause area. It displays detailed information about each charity, including its mission, programs, and financials.
- Donation platform: Integrate donation functionality into your platform, enabling users to make secure online donations to their chosen charities.
- Grant management system: Use the API to verify charity eligibility, track grant applications, and manage fund disbursements.
By using OrgHunter's Charity API, you can:
- Save time and resources: Access the data you need without collecting and maintaining it manually.
- Improve data accuracy: Rely on OrgHunter's comprehensive and up-to-date database.
- Enhance your application: Provide users with valuable tools and information to support their philanthropic goals.
Ready to Get Started?
Visit the Charity API documentation to sign up for a free account and start exploring the Charity API. With OrgHunter, you can easily integrate charity data into your applications and empower your users to make a difference.